֍
Learn how to use masked image generation techniques using StableDiffusionXLControlNet and StableDiffusionXLInpaint.
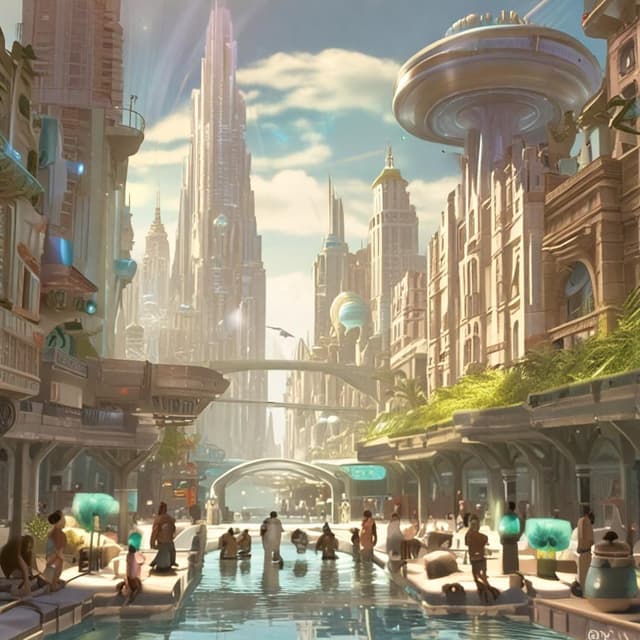
Generate illusions
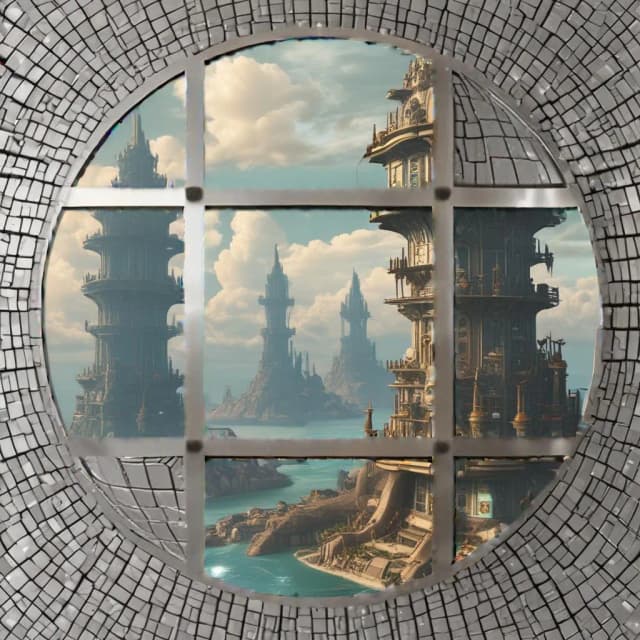
Edge variation and inpainting
We'll cover two workflows that use masked image generation:
- Generate illusions: StableDiffusionXLControlNet with the
illusion
method generates illusions incorporating the mask. - Edge variation and inpainting: StableDiffusionXLControlNet with the
edge
method generates variations of an image using edge detection, and StableDiffusionXLInpaint generates inside the mask.
First, initialize Substrate:
Here's the original image:
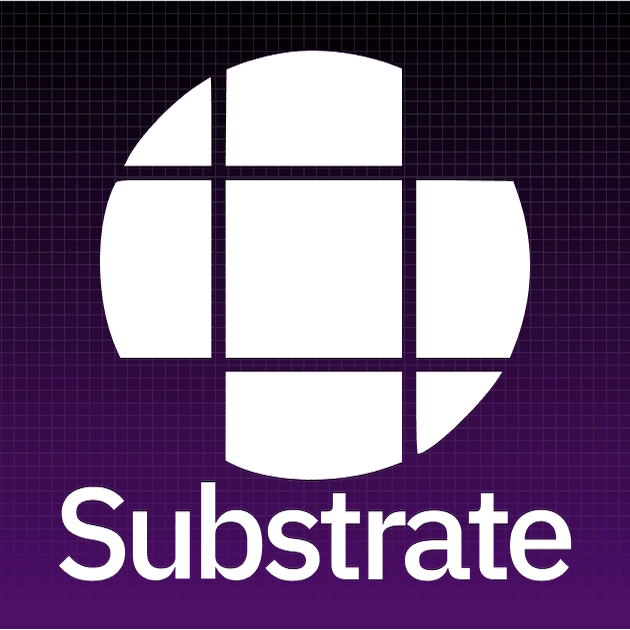
1. Generate illusions
This workflow uses RemoveBackground to generate a mask, followed by StableDiffusionXLControlNet with the illusion
method to generate a two images incorporating the mask into a view of the ocean from above:
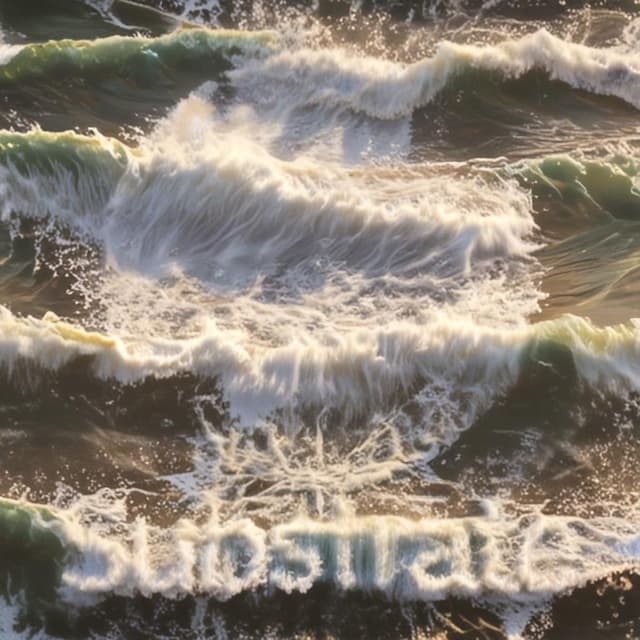
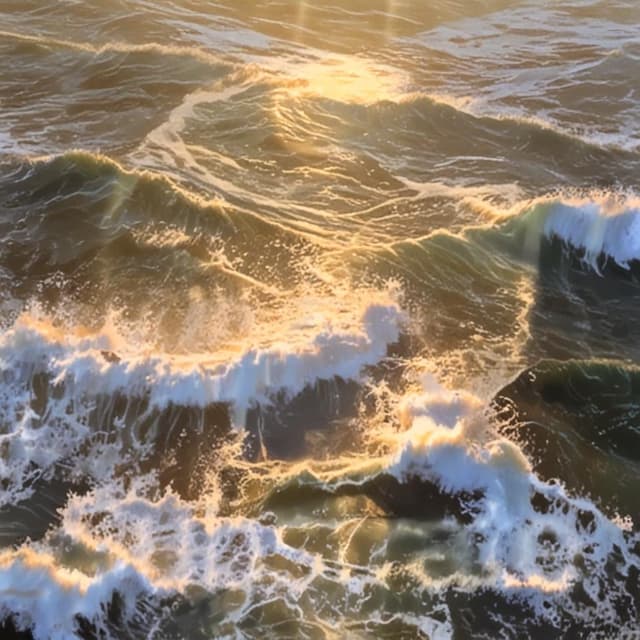
Experimenting with different prompts can produce striking results:
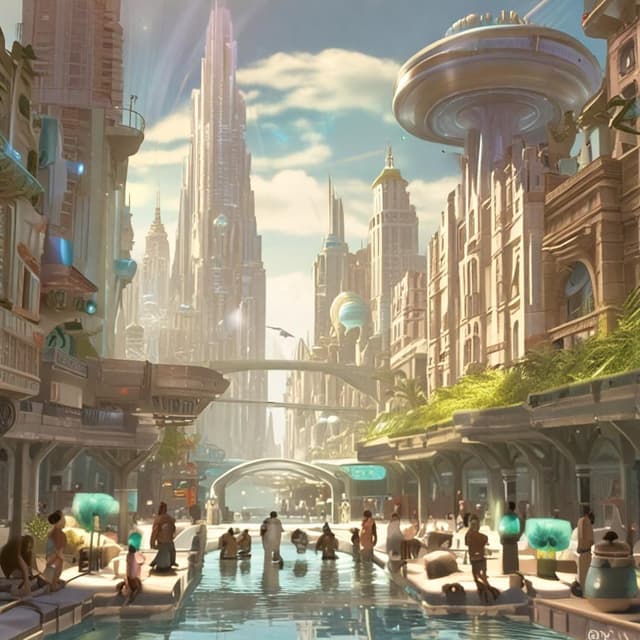
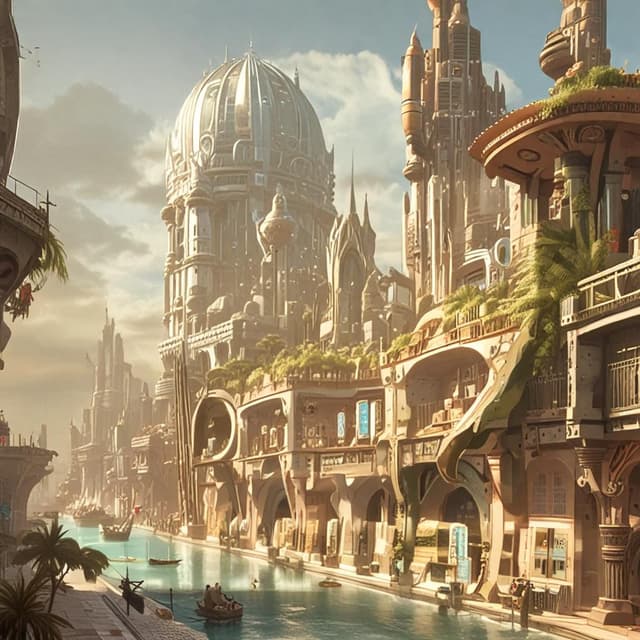
2. Edge variation and inpainting
This workflow uses StableDiffusionXLControlNet to generate a variation of the original, and StableDiffusionXLInpaint to generate a variation inpainting inside a mask.
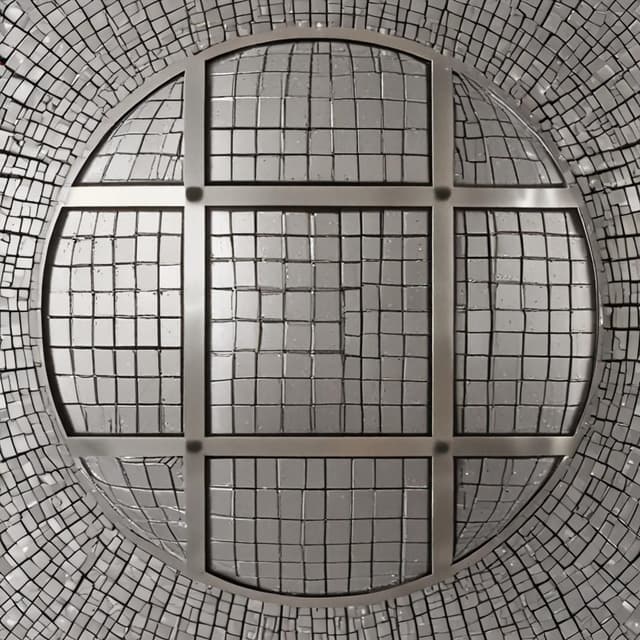
res.get(controlnet)
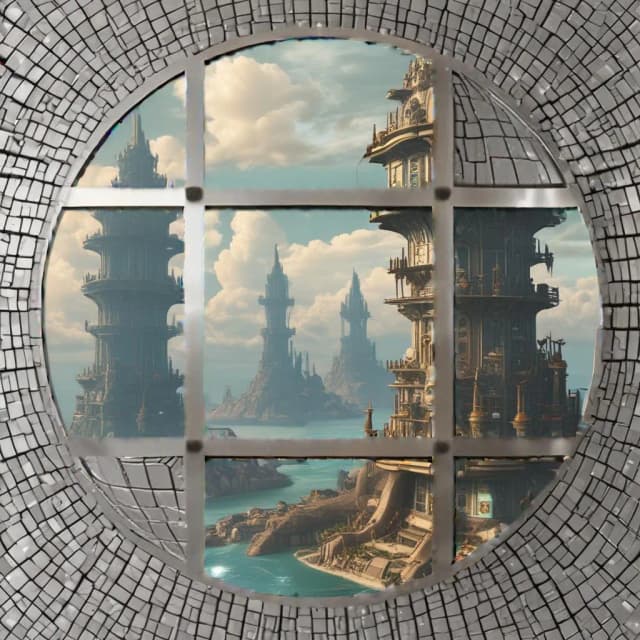
res.get(inpaint)